Mbeans(JMX) can be very useful to manage an application or any resource. ADF provides some inbuilt mbeans. These can be enabled by adding the below tags in web.xml
<listener>
<listener-class>
oracle.adf.mbean.share.connection.ADFConnectionLifeCycleCallBack
</listener-class>
</listener>
<listener>
<listener-class>
oracle.adf.mbean.share.config.ADFConfigLifeCycleCallBack</listener-class>
</listener>
<listener>
<listener-class>
oracle.bc4j.mbean.BC4JConfigLifeCycleCallBack</listener-class>
</listener>
Some times you might want to add custom Mbeans to control your application. You also might want to access these using your jrockit mission control. Lets understand this by take an instance of changing log levels of certain classes when you use log4j for logging using mbeans(JMX).
1. First you need an interface of the methods you want to expose.
public interface Log4jConfigMBean {
public String fetchLogLevel(String logger);
public String changeLogLevel(String level, String logger);
}
2. You need a class that implements the previously created Log4jConfigMBean and javax.management.MBeanRegistration
package com.test.backing;
import javax.management.MBeanRegistration;
import javax.management.MBeanServer;
import javax.management.ObjectName;
import org.apache.log4j.Level;
import org.apache.log4j.Logger;
public class Log4jConfig implements Log4jConfigMBean , MBeanRegistration {
public Log4jConfig() {
super();
}
public String fetchLogLevel(String name) {
if (name==null ||"".equals(name) )
{
return "invalid usage";
}
else
{
return "Log level of " +name +" is "+ (Logger.getLogger(name).getLevel()!= null ?Logger.getLogger(name).getLevel() :Logger.getRootLogger().getLevel());
}
}
public String changeLogLevel(String level, String name) {
if (name==null ||"".equals(name)|| level==null || "".equals(level) )
{
return "invalid usage";
}
else
{
Logger logger = Logger.getLogger(name);
logger.setLevel(Level.toLevel(level));
return "Log level of "+ name + " set to " +Logger.getLogger(name).getLevel();
}
}
public ObjectName preRegister(MBeanServer mBeanServer, ObjectName objectName) {
return null;
}
public void postRegister(Boolean boolean) {
}
public void preDeregister() {
}
public void postDeregister() {
}
}
3. We have to register these in someplace when the application starts. I chose initialize these using a ServletContextListener. You can use other methods too.
package com.test.backing;
import java.lang.management.ManagementFactory;
import javax.management.MBeanServer;
import javax.management.ObjectName;
import javax.naming.InitialContext;
import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener;
public class Log4jMBeanLifeCycleListener implements ServletContextListener {
public Log4jMBeanLifeCycleListener() {
super();
}
public void contextInitialized(ServletContextEvent servletContextEvent) {
try {
InitialContext ctx = new InitialContext();
MBeanServer mbs = ManagementFactory.getPlatformMBeanServer();
Log4jConfig mbean = new Log4jConfig();
ObjectName oname;
oname = new ObjectName("Log4j:type=Log4jConfig,name=RuntimeLog4jConfig");
mbs.registerMBean(mbean, oname);
} catch (Exception e) {
e.printStackTrace();
}
}
public void contextDestroyed(ServletContextEvent servletContextEvent) {
}
}
4. Add the ServletContextListener to the web.xml
<listener>
<listener-class>com.test.backing.Log4jMBeanLifeCycleListener</listener-class>
</listener>
5. Testing the mbeans.
a. Make sure you have enabled JMX on the weblogic server. In Jdeveloper you can add them to the Run Configurations
-Dcom.sun.management.jmxremote -Dtangosol.coherence.management=all -Dtangosol.coherence.management.remote=true -Dcom.sun.management.jmxremote.port=7772
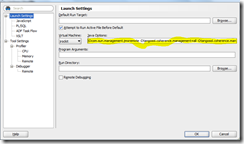
b. Start jrockit. You can now see the new Management Extension. You can change the log level in runtime.
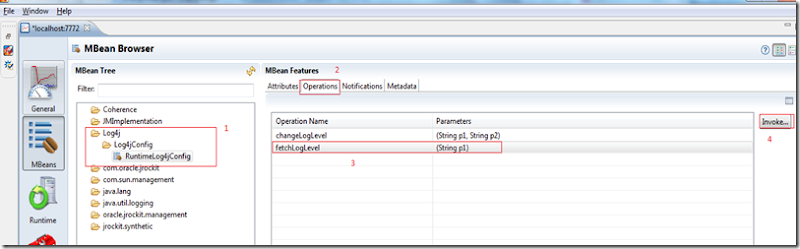